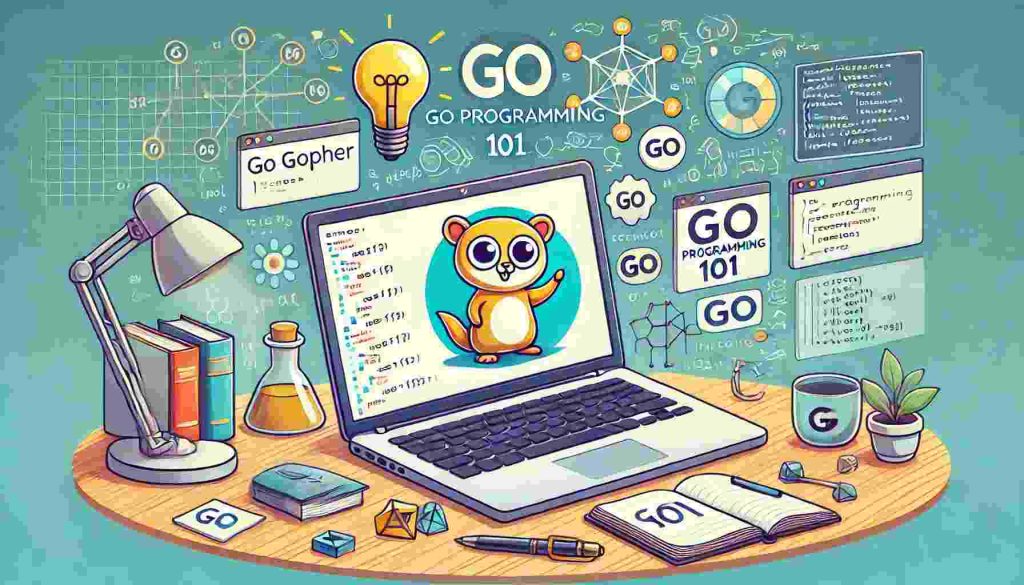
Go Programming 101: Your First Steps into the Go Programming Language
As we know that in today’s world programming languages are very vast and dynamic in nature so the Go language is also one of the powerful, efficient and global accepted language by developers. Developed by Google, by two engineers in 2007 and then released in 2009, Go, also known as Golang, is intended to address the issues of modern software development while, at the same time, maintaining high speed and coping well with the increasing volumes of data. In this article, I will show you how to get started with Go Programming 101, from the ground up, giving you the rudimentary knowledge on how to code using Go.
What is Go Programming?
Go is the open source language that is designed to be as easy to use as Python-like languages, but is as fast as C and C++ and as safe as them. It was designed with three primary goals: These approaches include; efficiency, simplicity and reliability. It is specially suitable for the development of concurrent, distributed applications that is why GO is used in Cloud computing, microservices and large-scale backend systems.
Key Features of Go
Simplicity: Go’s syntax is rather minimalistic and well-arranged; there are no unnecessary frills that can complicate a programmer’s work. This also implies fewer line of codes and as is usually the case with such, there is limited allowance for code errors.
Concurrency: The goroutines & channels make Go inherently capable of concurrent programming & therefore suitable for creation of applications that could perform multiple tasks.
Performance: Still, being a statically typed, compiled language, Go provides very high performance rates, comparable with such lower-level languages as C.
Garbage Collection: Go, for instance, comes with a garbage collector which helps perform memory management autonomously thus reducing the incidences of memory leaks and other related pitfalls.
Cross-Platform: Go compiles into native binaries for all the platforms which include Windows, MacOS X, and Linux among others.
Why Learn Go Programming?
So, learning Go is beneficial because after its study one opens a vast range of opportunities, especially for the ones who are keen on system-level programming, cloud services, and backend development.
Here are a few reasons why Go Programming 101 is an excellent starting point for beginners and experienced developers alike:
Industry Adoption: Some of these companies which have adopted Go include Google, Dropbox, and Uber among others, and this has gone a long way in proving the reliability of the language due to the critical parts of their infrastructure implemented in Go.
Growing Community: It grows at a very fast pace which tells you that there are many developers, many resources, libraries and tools out there to support a Go enthusiast.
Career Opportunities: The demand for go developers is high particularly in organizations that deal with issues of cloud computing, DevOps among others in designing scalable systems.
Getting Started with Go Programming
1. Setting Up Your Go Environment
Before developing anything in Go, you will have to know how to configure your development environment. Follow these simple steps to get started:
Install Go: Visit Go official site, found at golang. org and download the latest version of the Go compiler for your operating system they are as follows. Installation is simple and information regarding the same is well given on the website of the company in question.
Set Up Your Workspace: Go needs the certain environment arrangement. Typically, your Go workspace will consist of three directories: src for source files, pkg for compiled package objects and bin for compilable binaries. The $GOPATH environment should point to the root directory of your Go workspace.
Verify Installation: Open your terminal, if you are on Mac or terminal in windows or command prompt and type go version. If Go is properly installed, you will get the version of the Go you installed in your system.
2. Writing Your First Go Program
Now it is time to write the first Go program and let’s start. In this example, we will make the usual “Hello, World!” program.
- Step 1: Create a New Directory
bash
mkdir hello
cd hello
- Step 2: Write the Code Inside the
hello
directory, create a new file namedmain.go
and open it in your preferred text editor. Write the following code:gopackage main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
- Step 3: Run the Program Save the file and return to your terminal. Run the program with the following command:
go
go run main.go
If everything is set up correctly, you should see the output:
Hello, World!
3. Understanding the Basic Structure of a Go Program
The “Hello, World!” program introduces several key concepts in Go:
- Package Declaration: The
package main
line indicates that this file belongs to themain
package, which is the starting point for executable Go programs. - Imports: The
import "fmt"
line tells Go to include thefmt
package, which contains functions for formatted I/O operations. - Functions: The
func main()
is the entry point for the program. Themain
function is where execution starts.
4. Exploring Go’s Syntax and Features
Variables and Data Types
Go is a statically typed language, which means every variable must have a specific type. You can declare variables using the var
keyword or the shorthand :=
operator.
var name string = "Go Programming"
age := 10
Control Structures
Go supports standard control structures like loops (for
), conditionals (if
, else
), and switch cases.
if age > 5 {
fmt.Println("Go is maturing fast!")
} else {
fmt.Println("Go is still young.")
}
Goroutines and Concurrency
One of Go’s standout features is its support for concurrency through goroutines. A goroutine is a lightweight thread managed by the Go runtime.
go sayHello()
fmt.Println("This runs concurrently!")
5. Building and Installing Go Programs
Go makes it easy to compile and install programs. To build your program into an executable, simply use the go build
command:
go build main.go
This will produce a binary named main
(or main.exe
on Windows) in your current directory. You can run this binary directly:
./main
6. Leveraging Go’s Standard Library
Go comes bundled with a very rich standard library that handles a lot of topics such as file operations, HTTP request processing, data manipulation operations and a lot more. A good preparation for a standard library is necessary as a programmer progresses in Go programming.
Tips for Mastering Go Programming 101
Practice Regularly: As with any language; he practice is key. First, using Go, work on simple missions and then try to tackle other complicated assignments.
Read Go Documentation: In general, Go’s official documentation is clear and … it covers almost any topic one may think of concerning the language. It should become a practice to read the documentation in other to grasp new ideas or changes made on the tool.
Join the Go Community: Here are some ways through which you can start your interaction with the Go community. Go to discussion boards, attend meetings or donate your time to open source projects. This is a place where one is privileged to learn and at the same time expanding his or her network.
Conclusion
This “Go Programming 101” is your ticket to taming a language that is quickly becoming a standard in software development space. As a language with primary design proficiencies in simplicity, efficient execution, and robust simultaneous computing, Go is ideal for construction by developers who seek to create scalable applications of high proficiency. Thus by following this guide and practicing regularly you are in the right path and would be able to master Go programming very soon. Hence there is no better time than the present, and it is time for you to begin your journey, and start coding with Go!

Read Dive is a leading technology blog focusing on different domains like Blockchain, AI, Chatbot, Fintech, Health Tech, Software Development and Testing. For guest blogging, please feel free to contact at readdive@gmail.com.